How to Use Python to Create and Implement Microservices
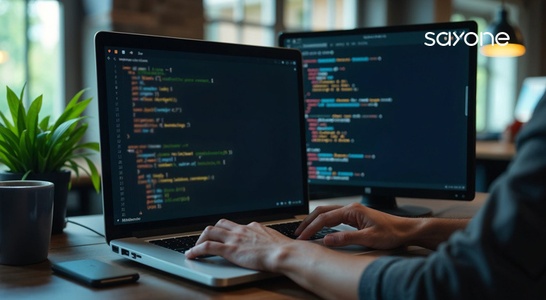
Share This Article
Table of Contents

Subscribe to Our Blog
We're committed to your privacy. SayOne uses the information you provide to us to contact you about our relevant content, products, and services. check out our privacy policy.
Python stands as a leading programming language for microservices development, with data showing its strong position in software architecture.
Industry research shows that 90% of companies now use or plan to use microservices, while Python ranks in the top three languages chosen by developers, with 31% selecting it as their primary tool for building microservices.
The combination of Python's clear syntax, rich library collection, and proven frameworks like Flask and FastAPI helps teams build and scale microservices effectively. Companies using this approach report concrete benefits: 28% better application performance and 26% higher staff productivity.
These improvements stem from Python's built-in features that support code readability and maintenance, especially valuable when multiple teams work on separate services.
Major platforms including Instagram, Spotify, and Netflix demonstrate Python's capabilities in large applications. Python excels in RESTful API development, asynchronous programming, and works well with Docker and Kubernetes for containerization.
The practical success, combined with measurable performance gains, shows why Python continues to grow as a preferred choice for modern microservices.
Getting Started with Python Microservices
Python microservices create scalable, maintainable, and adaptable software architectures. Breaking down complex applications into smaller, independent services allows businesses to develop, deploy, and scale components independently, leading to better performance and reliability.
To Do #1: Setting Up the Development Environment
A well-configured setup forms the base of successful microservices development. Begin by creating a virtual environment for clean dependency management.
IDEs like PyCharm or VS Code provide excellent microservices development features, including automated debugging and testing tools.
Implement Git-based version control practices from day one.
To Do #2: Choose Python Libraries and Frameworks
Python offers specialized frameworks for microservices. FastAPI excels with async capabilities and automatic OpenAPI documentation, while Flask delivers simplicity and adaptability.
The above image shows microservices architecture where a web browser interacts with client-side rendering through an Nginx load balancer. The system comprises three Docker-containerized Django REST APIs (v1-v3), each connecting to separate applications (Vote, Polls, Choice) with isolated databases. The entire setup integrates with third-party services at the bottom layer.
Django REST framework suits complex architectures with comprehensive features. Nameko helps with service-to-service communication, while PyMS provides built-in monitoring tools.
To Do #3: Basic Project Structure
A clear, organized structure supports maintainable microservices. Apply domain-driven design by arranging services around business functions. Each service needs its own database to maintain independence. Follow this tested structure:
Main Components:
- Service Layer (Business Logic)
- API Layer (External Communication)
- Data Layer (Storage & Retrieval)
- Utility Services (Shared Functionality)
Advanced Considerations
Add service discovery and registration for scaling and load balancing. Include circuit breakers like PyBreaker to manage service failures. Use message queues like RabbitMQ or Apache Kafka for asynchronous service communication.
To Do #4: Security and Monitoring
Add JWT-based authentication and rate limiting to secure services. Monitor service interactions with tracing tools like Jaeger or Zipkin. Set up comprehensive logging with ELK stack (Elasticsearch, Logstash, Kibana) for better system visibility and problem-solving.
Maintain detailed API documentation and implement versioning practices to support long-term service maintenance.
Building Your First Microservice with Python
Python microservices change application architecture, making systems more scalable and maintainable. Companies like Netflix, Uber, and Amazon apply this method to handle millions of requests daily.
Step 1: Setting Up the Foundation
Begin with your development setup. Create a virtual environment and install your preferred python framework suited for microservices. Your architecture requires specific planning for service registry integration and API gateway configuration. Service registry manages discovery tasks, API gateways direct request routing, and load balancers spread traffic across your services.
Step 2: Designing RESTful Endpoints
Clear API design underpins your microservice success. Use REST principles with standard HTTP methods and status codes. Create endpoints that match business needs instead of technical functions.
Start with versioning to keep backward compatibility. Your endpoint design should address resource naming, request/response formats, and authentication methods for secure API operations.
What's the difference between REST API and RESTful API in microservices?
While often used interchangeably, REST API refers to any API that follows REST architectural guidelines, while RESTful API fully implements all REST constraints including HATEOAS (Hypertext As The Engine Of Application State). RESTful APIs in microservices provide better scalability and loose coupling between client and server.
Checkout the Top 5 Technologies to Build Microservices Architecture
Step 3: Implementing Communication Patterns
Service communication determines system dependability. Combine synchronous and asynchronous patterns using message queues like RabbitMQ or Apache Kafka. Event-driven approaches keep services independent.
Message queues support reliable data flow between services, while event-driven patterns help with scaling and error management.
Step 4: Error Handling and Monitoring
A complete microservices production setup needs circuit breakers and fallback mechanisms, contextual logging, and advanced metrics monitoring through Prometheus-Grafana integration.
The system includes distributed tracing with Jaeger or Zipkin, automated health checks, performance metrics, and active security monitoring with threat detection capabilities. These features combine to maintain system reliability, maintainability, and security.
These methods build dependable, well-performing systems that adapt to growth while staying stable. Each part works together in a microservice structure that supports business needs and technical quality.
Step 5: Security and Authentication
Strong security measures are essential in microservices architecture, safeguarding sensitive data and enabling reliable service interactions. An effective security strategy includes multiple protection layers while maintaining consistent system performance.
Step 6: JWT Authentication Implementation
JSON Web Tokens provide a stateless, scalable approach for authentication between microservices. Organizations implement JWT for secure information transmission through digitally signed JSON objects, which proves highly effective in microservices setups.
The process includes creating time-bound tokens, incorporating user claims, and applying cryptographic signatures for data integrity. A well-executed JWT authentication system creates a secure foundation for service-to-service communication while supporting system growth.
Read More on the Best Practices to Secure Microservices Architecture
Step 7: API Security Installation
Modern API security requires a sophisticated multi-layered method combining authentication, authorization, and encryption mechanisms. Transport layer security via TLS 1.3 handles data encryption during transmission, while OAuth 2.0 and JWT manage identity verification.
Security Components:
- Access management through role-based and attribute-based systems
- Multi-layer protection implementation
- Immediate threat detection and response
- Active security monitoring and logging
Step 8: Rate Limiting Architecture
Rate limiting defends microservices infrastructure using specific implementation methods and safety features. The setup uses traffic-based rate controls, token bucket and leaky bucket algorithms, Redis-based request tracking, load-based limit changes, and multi-node rate limiting synchronization.
These tools help block DoS/DDoS attacks, balance resource use, keep services running, and manage high-traffic periods across the system.
Step 9: Testing and Deployment
Testing and deployment serve as essential building blocks in creating reliable microservices, where individual components and the complete system work together while maintaining high availability.
Unit Testing Excellence
Unit testing acts as the quality foundation for microservices. Python's pytest framework offers advanced capabilities for testing individual service components. Current practices focus on behavior-driven development (BDD) and test-driven development (TDD) methods, validating each service meets its specifications before deployment.
Integration Testing Mastery
Integration testing checks the interactions between microservices, examining API contracts and data flow. Consumer-driven contract testing confirms services fulfill consumer needs while keeping backward compatibility. Tools like Postman and SoapUI run automated API testing, while Pact helps manage service contracts.
Read more on Testing Microservices – A Complete Guide
How do you handle database dependencies in microservices integration testing?
Integration tests use in-memory databases to mirror real database interactions without full deployment costs. This method allows quick test execution while maintaining realistic data scenarios. TestContainers helps create isolated database instances for testing, ensuring dependable and repeatable results.
Step 10: Docker Containerization
Docker containers create matching setups across development and production stages. Each microservice operates in its isolated container, stopping dependency conflicts and ensuring exact deployments. Container orchestration with Kubernetes handles scaling, load balancing, and service discovery, improving deployment reliability.
Step 11: CI/CD Implementation
Modern CI/CD pipelines automate testing and deployment steps. Jenkins or GitLab CI detect code changes, starting automated tests and containerization. After successful testing and deployment using different continuous Integration tools run updates using blue-green or canary deployment methods to reduce downtime and risk.
Pipeline Architecture:
- Code validation and unit testing
- Container building and security scanning
- Integration testing in staging
- Production deployment with monitoring
Microservice Performance Optimization How to do it
Optimizing microservices affects user experiences and system efficiency directly. When Python microservices handle increasing loads, proper optimization strategies help your business succeed.
1. Strategic Caching
Response time impacts sales and user retention. Redis and Memcached integration with Python microservices reduces database loads and API response times. Using both client-side and service-side caching patterns cuts latency by 80% while maintaining data consistency.
2. Intelligent Load Balancing
Nginx and HAProxy provide advanced algorithms for request distribution. Server-side load balancing with health checks and automatic failover keeps systems running. AWS, Azure, and Google Cloud solutions add SSL termination and DDoS protection for better security.
How does intelligent load balancing differ from traditional load balancing in microservices architecture?
Intelligent load balancing uses data-driven algorithms and real-time analytics for routing, unlike basic distribution methods. It analyzes server health, response times, and resource usage to direct traffic. This approach enables auto-scaling, early problem detection, and improved reliability in microservices systems.
3. Performance Monitoring
Prometheus and Grafana track key metrics effectively. Monitor request latency, error rates, and resource usage through custom dashboards. Create alerts for unusual patterns and watch system health metrics in real-time.
Learn more about How to Improve Performance of Microservices
Why Is SayOne the Right Partner for Python Microservices Solutions?
Are you struggling with the complexities of building and deploying microservices? Many businesses face challenges in creating scalable, maintainable, and well-structured microservices architectures.
At SayOne, we specialize in addressing these challenges with our expertise in Python-based microservices development.
Our team has a proven track record of delivering reliable solutions tailored to diverse business needs. From startups to enterprises, we’ve helped organizations improve their operations using cutting-edge technologies like Flask, Django, and Kubernetes.
As a leading outsourcing partner, we ensure timely delivery, high-quality code, and flexible engagement models to meet your unique requirements.
Let us help you bring your vision to life. Contact with SayOne today to build microservices that drive growth and efficiency!
Share This Article
Subscribe to Our Blog
We're committed to your privacy. SayOne uses the information you provide to us to contact you about our relevant content, products, and services. check out our privacy policy.
Related Articles
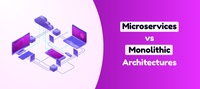
Microservice Architecture
A Complete Guide For Microservices Vs. Monolithic Architectures