React Design Patterns for 2024 Projects (Complete Guide)
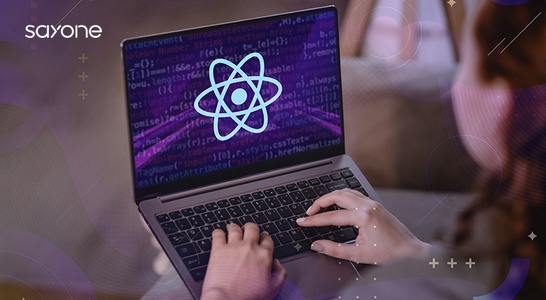
Share This Article
Table of Contents

Subscribe to Our Blog
We're committed to your privacy. SayOne uses the information you provide to us to contact you about our relevant content, products, and services. check out our privacy policy.
React design patterns are tools that help developers build efficient and scalable applications. They're not just techniques but roadmaps to simplify app development. These patterns address common problems, allowing for quicker and more reliable solutions. They're divided into several categories, each serving a unique purpose in the development process.
Why Use Design Patterns?
Design patterns in React play a crucial role in the app development lifecycle. They provide tested solutions to common issues, enabling developers to avoid reinventing the wheel. For instance, the Context API and the Compound Components pattern allow for effective state and logic sharing among components, avoiding prop drilling and making the code cleaner and more maintainable.
Categories of Design Patterns
- State Management: Patterns like the Provider pattern help manage global state across the component tree, making it easier to pass data around without cluttering the component hierarchy.
- Data Fetching and Management: Using custom hooks for data fetching, such as fetching characters in an app, exemplifies how React patterns can simplify asynchronous operations and state management related to data retrieval.
- UI Composition: Compound Components and the Higher-Order Components (HOCs) pattern allow for more flexible and reusable UI structures. These patterns help in composing complex UIs from simpler, reusable components.
- Functional Enhancements: Patterns like Render Props and HOCs are also used to enhance component functionality without altering their core logic, providing a means to share logic and state across components seamlessly.
Here are the Best React Design Patterns You Should Know in 2024
Component Design Patterns
Understanding and applying certain design patterns can greatly improve your code's structure and maintainability when crafting React applications. Let's break down some key component design patterns you might find useful for your projects.
Higher Order Components (HOC)
HOCs enhance components with additional functionality or data without modifying their code. This pattern is particularly helpful for reusing logic across different components, such as handling user authentication, data fetching, or applying styles.
Use Cases:
- Data Fetching: Wrapping components to inject data from an API.
- User Authentication: Conditionally rendering components based on user authentication status.
- Style Enhancement: Applying common styling or themes across multiple components without repeating code.
Render Props Pattern
The render props pattern allows for more dynamic component composition by using a function prop that components can use to know what to render. This pattern facilitates sharing code between components and enables developers to create more customizable and flexible UI components.
Use Cases:
- Sharing Stateful Logic: Allowing multiple components to share and use the same stateful logic without higher-order components.
- Conditional Rendering: Providing a mechanism for components to dynamically determine how and what they render based on certain conditions.
- Reusable Animation Logic: Sharing animation logic across components, where each component can use its own rendering logic with shared animation states.
Compound Components
Compound components allow complex components to be broken down into smaller, manageable pieces that manage their state and interactions internally. This pattern is useful for creating components with a shared state that need to communicate and manage logic collectively.
Use Cases:
- Building UI Toolkits: Designing complex UI elements, such as accordions, tabs, and dropdowns, that require multiple components to work together seamlessly.
- Form Libraries: Creating a form library where inputs, labels, and error messages are separate components but need to share validation logic and form state.
- Configurable Layout Components: Designing layout components that need to be highly configurable but maintain a consistent structure and state management.
Context API Utilization
The Context API allows for easy state sharing across an entire app without prop drilling, making it simpler to pass down data and callbacks through the component tree. It's particularly useful for a global state that needs to be accessed by many components at different nesting levels.
Use Cases:
- Theme Switching: Allowing components to access and render based on the current theme (dark mode/light mode) without passing props down every level.
- Localization and Internationalization: Sharing locale-specific data, such as translations across all components in an application.
- Managing User Sessions: Keeping track of user authentication state and permissions across all components that need to conditionally render based on user status.
Structural design patterns
Structural design patterns in React focus on how components are organized and interact with each other to simplify relationships and improve efficiency. By implementing these patterns, developers can ensure their applications are scalable, maintainable, and easy to understand. Let's explore three key structural design patterns:
Container-Presentational Pattern
This pattern separates the concerns of how things look (Presentational components) from how they work (Container components). Presentational components are concerned with rendering the UI, whereas Container components handle fetching data, state management, and passing data to the presentational components. This separation enhances reusability and simplifies testing.
Use Cases:
- Separating Logic from UI: Building UI components like buttons, inputs, and forms without embedding business logic.
- Reusable Containers: Implementing containers that fetch and manage data, which can be used with various presentational components.
- Simplifying Testing: Testing components independently; UI components can be tested with static data, and containers can be tested for their behavior and data processing.
Provider-Consumer Pattern
This pattern uses the Context API to pass data through the component tree without having to manually pass props at every level. It allows for more efficient state management across widely separated components and simplifies the component tree.
Use Cases:
- Theme Management: Providing a theme context to style components consistently across the application without prop drilling.
- User Authentication: Managing user authentication status and information, making it accessible to all components that require it.
- Global State Management: Sharing global states like user settings, application configuration, or UI states without relying on global state management libraries.
Checkout React JS vs. React Native for Mobile App Development
Hooks Pattern: useState, useEffect, Custom Hooks
Hooks offers a way to use state and other React features without writing a class. useState allows for state management in functional components, ‘useEffect’ manages side effects in functional components, and custom Hooks enable reusing stateful logic across multiple components.
Use Cases:
- State Management in Functional Components: Using ‘useState’ to add stateful behavior to functional components, making them as capable as class components.
- Performing Side Effects: Using ‘useEffect’ for data fetching, subscriptions, or manually changing the DOM in React components.
- Reusable Logic: Creating custom Hooks to share common functionality between components, such as form handling logic, fetching data, or subscribing to an external data source.
Behavioral design patterns
Behavioral design patterns in React focus on improving the communication between components and the way they manage state and side effects. These patterns help in creating a more predictable flow of data and side effects, making the application easier to understand and maintain. Let's delve into three such patterns:
State Reducer Pattern
The State Reducer Pattern gives more control over state updates by allowing the logic of state changes to be customized from outside the component. This pattern is beneficial for creating highly customizable and controllable components, as it externalizes the state logic, making the internal component logic simpler and more predictable.
Use Cases:
- Customizable State Logic: Allowing library consumers to modify the state update logic without changing the component's internal code.
- Complex State Management: Managing complex state logic that depends on previous states in a more predictable manner.
- Controlled Components: Creating components that need to be tightly controlled from the outside, offering more flexibility to the developers.
Strategy Pattern
The Strategy Pattern involves defining a family of algorithms, encapsulating each one, and making them interchangeable. This pattern lets the algorithm vary independently from clients that use it. In the context of React, it can be used to dynamically change the behavior of a component based on its props or state.
Use Cases:
- Dynamic Rendering: Switching between different rendering strategies based on certain conditions, such as user roles or feature flags.
- Form Validation: Applying different validation strategies for form inputs based on the form's context or configuration.
- Data Fetching: Changing the data fetching logic dynamically, for example, based on the network status or the user's preferences.
Observer Pattern
The Observer Pattern is a behavioral design pattern where an object, known as the subject, maintains a list of its dependents, called observers, and notifies them of any state changes, typically by calling one of their methods. It's used in React to create a reactive and efficient way to update components when state or data changes.
Use Cases:
- State Synchronization: Synchronizing state across multiple components without lifting state up or using Context API.
- Event Handling: Creating a centralized event handling mechanism where multiple components can listen to and react to events.
- Data Streaming: Implementing a data streaming pattern where components can subscribe to data updates and react accordingly.
Advanced React patterns
Advanced React patterns focus on sophisticated techniques that enhance component reusability, state management, and the overall architecture of React applications. These patterns provide powerful solutions for common advanced use cases, enabling developers to build more flexible, maintainable, and scalable applications. Let's explore three such patterns:
Compound Components with Context API
This pattern uses the Context API to manage the state and behavior of interconnected components without prop drilling. It allows components to share states and functions through a context, making the parent-child communication cleaner and more manageable. This pattern is particularly useful for building complex component libraries that require shared state or behavior.
Use Cases:
- UI Toolkits and Libraries: Creating complex UI components, such as tab systems or dropdown menus, where multiple components need to share state logic.
- Form Libraries: Building form components where form elements like inputs, labels, and error messages need to access form state or validation logic from a parent form component.
- Configurable Component Systems: Designing a system of components that can be easily configured and reconfigured by the developer using them, with shared state or behavior.
Checkout Top 7 JavaScript Libraries
Controlled and Uncontrolled Components
This pattern differentiates between components that manage their own state (uncontrolled) and components whose state is managed by parent components (controlled). It provides flexibility in handling form inputs and other components, allowing for both internal state management and external control.
Use Cases
- Form Handling: Switching between controlled and uncontrolled inputs based on the need for flexibility or tight control over form data.
- Third-party Integrations: Integrating with third-party components where you might not have control over the component's internal state.
- Dynamic Forms: Creating dynamic forms where the need to add, remove, or modify inputs on the fly dictates whether components should be controlled or uncontrolled.
Custom Hooks for Reusable Logic
Custom Hooks allow for the extraction and reuse of stateful logic across components without the need for higher-order components or render props. This pattern simplifies code, improves readability, and makes state and side effects management more modular and testable.
Use Cases:
- Data Fetching: Creating a ‘useFetch’ hook to encapsulate the logic for fetching data from an API, making it reusable across components.
- Form Logic: Implementing custom hooks like ‘useForm’ to manage form state, validation, and submission, reducing boilerplate in form components.
- Event Listeners: Crafting hooks like ‘useEventListener’ to handle adding and removing event listeners, encapsulating the logic for dealing with browser events in a reusable way.
If you're looking to apply these patterns in your projects and need professional assistance, SayOne is here to help. As a dedicated React development firm, SayOne offers expertise in building high-quality, scalable applications using React. Our Team is well-versed in the latest React techniques and committed to delivering top-notch solutions.
Reach out to SayOne for your React development needs and take the next step towards creating outstanding applications.
Share This Article
Subscribe to Our Blog
We're committed to your privacy. SayOne uses the information you provide to us to contact you about our relevant content, products, and services. check out our privacy policy.